

How to: Suppress violations in rule reports |
Sometimes the analysis reports potential issues which should not appear in the reports.
Currently only the suppression of warnings in assemblies and in XML SharePoint code (e.g. Features) are supported. Suppression of warnings in CSS, JavaScript or HTML code are currently not possible.
- Suppress warnings in Assemblies (.NET code)
- Suppress warnings in XML code
- Suppressed warnings shown in reports
Suppress warnings in Assemblies (.NET code)
To suppress SPCAF warnings in assemblies SPCAF supports the attribute "System.Diagnostics.CodeAnalysis.SuppressMessage" of .NET.
The attribute takes 2 arguments for category of the suppressed rule and for the checkid.
The checkid (second parameter) can have a short form (only Id) and a long form (Id and name of rule).
Optional arguments which are interpreted by SPCAF is Justification. All other arguments (MessageId, Scope, Target) are currently not evaluated by SPCAF.
Important: The SuppressMessage attribute is a conditional attribute which is included in the IL metadata of your managed code assembly only if the CODE_ANALYSIS compilation symbol is defined at compile time.
Suppress warnings for a method
The following code snippet shows how the suppress warnings for rule "SPC050235 ApplyLockForCachingObjects" for a method. It also show how to provide a justification for the suppression.
In this case all warnings for the given rule in this method are suppressed which could lead to unexpected results if only a single warning should be suppressed.
using System.Diagnostics.CodeAnalysis;
...
namespace SuppressExample
{
public class ApplyLock
{
[SuppressMessage("SPCAF.Rules.BestPractice", "SPC050235:ApplyLockForCachingObjects", Justification = "ApplyLock removed.")]
public void MethodWithoutApplyLock()
{
...
}
}
}
Suppress warnings for a whole class
The following code snippet shows how to suppress all warnings for rule "SPC050235 ApplyLockForCachingObjects" for a whole class.
using System.Diagnostics.CodeAnalysis;
...
namespace SuppressExample
{
[SuppressMessage("SPCAF.Rules.BestPractice", "SPC050235:ApplyLockForCachingObjects")]
public class ApplyLock
{
...
}
}
Suppress warnings for a whole assembly
The following code snippet shows how to suppress all warnings for rule "SPC050235 ApplyLockForCachingObjects" for a whole assembly in file "AssemblyInfo.cs".
[assembly: AssemblyCompany("MyCompany")]
[assembly: AssemblyTitle("MyCompany.Intranet")]
...
[assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("SPCAF.Rules.BestPractice", "SPC050235:ApplyLockForCachingObjects")]
Suppress warnings in XML code
Since version v5.3 of SPCAF it is possible to suppress messages in SharePoint XML code, e.g. for Features, ContentTypes etc. See sample below:
<!-- "SuppressMessage":{"rule":"SPC015108:DeclareAllRecommendedAttributesInFields","justification":"Intentionally ignored"} -->
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<Field
Group="MyCompany.Intranet Columns"
DisplayName="InternalField Name"
ID="{C64E738E-C093-495C-9B32-BB944948D82E}"
SourceID="http://schemas.microsoft.com/sharepoint/v3"
StaticName="InternalField"
Name="InternalField" >
</Field>
<!-- "SuppressMessage":{"rule":"SPC015103:DefineUniqueFieldId","justification":"False positive"} -->
<Field
Group="MyCompany.Intranet Columns"
DisplayName="HiddenPageField Name"
ID="{32E36ECB-69DF-46B0-9FC3-D4C8A33C4EFC}"
SourceID="http://schemas.microsoft.com/sharepoint/v3"
StaticName="HiddenPageField"
Name="HiddenPageField" >
</Field>
</Elements>
The suppression comment is applied to the XML node AFTER the comment.
In contrast to the suppression in assemblies the directive "CODE_ANALYSIS" is not necessary for XML code suppressions.
Scope of Suppressions in XML
The suppression in the XML code is applied to the loaded object in SPCAF, e.g. a Feature instance. This means a suppression for a Feature which targets ContentTypes is then applied to ALL ContentTypes in this Feature, although they are not part of the XML file. See sample below:
<!-- "SuppressMessage":{"rule":"SPC045201:DeclareAttributeInheritsInContentType","justification":"Attribute 'Inherits' not wanted"} -->
<Feature xmlns="http://schemas.microsoft.com/sharepoint/"
Id="3d7f4c47-fa71-412c-91bb-da8e7552daab"
Title="MyFeature"
...
<ElementManifests>
<ElementManifest Location="ContentTypes\Elements.xml" />
...
</ElementManifests>
</Feature>
In sample above the suppression targets ContentTypes but it is added to the Feature. During the analysis the suppression is then applied to all ContentTypes in this Feature.
Suppressed warnings shown in reports
Note: The suppression of rule violations does not disable a rule completely. The rule is still executed during analysis but a rule violation is not counted in the number of errors or listed in the code quality reports as violations. But to allow tracability of suppressions the code quality report shows a list of all suppress violations (since SPCAF version 5.3). See screenshot below from a code quality report (HTML).
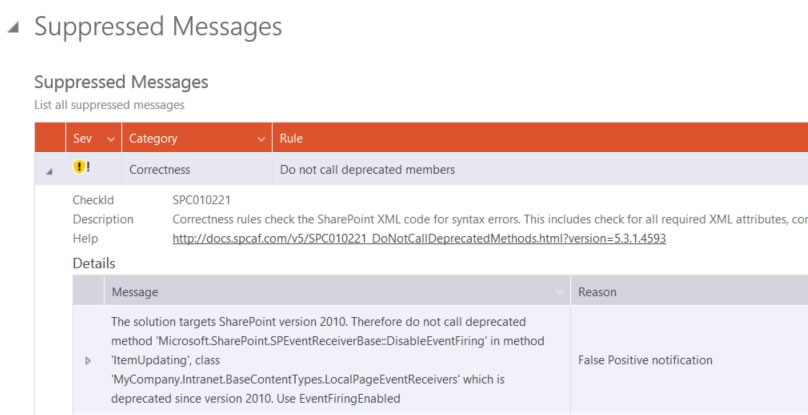